Generate same 3DES / AES-128 / AES-256 encrypted message with Python / PHP / Java / C# and OpenSSL Posted on May 26, 2017 by Victor Jia 2017/6/5 Update: Added C# implement.
- Generate Aes 256 Key Python 3.7
- Generate Aes 256 Key Python 3.5
- Generate Aes 256 Key Python Download
- Generate 256 Bit Aes Key Python
- Run import pyaes, pbkdf2, binascii, os, secrets # Derive a 256-bit AES encryption key from the password password = 's3cr3t.c0d3' passwordSalt = os.urandom (16) key = pbkdf2.PBKDF2 (password, passwordSalt).read (32) print ('AES encryption key:', binascii.hexlify (key)).
- AES 256 Encryption in Python. In my last post I left off after the key expansion portion of the algorithm. The next step is to carry out the encryption of the input data. First, the input data is split into a 4x4 matrix called the state matrix. The AES encryption operations work on this matrix.
Released:
Encryption has never been so easy!
Project description
CryptIt — is a simple and powerful cross-platform encryption tool, which can be used to protect your data from other people (like NSA, Government, Illuminati, big bro and so on) in the easiest possible way.
Cryptographic security of encrypted files is based on Advanced Encryption Standard (AES) algorithm in CBC mode with a key of 256-bits length.
To use cryptit you need to install python and pip on your personal computer, generate strong session password (for this step you can read useful article on xkcd site, it’s very important) and then read following instructions bellow.
How does it work?
You choose the mode in which the program would be launched [encryption or decryption] and pass a path to target file or directory as an argument. After that, you generate strong password and type that password in the program. Then cryptit calculates hash (SHA-3 256) of your password and uses it as key for AES-256 in CBC mode.
Encryption has never been so easy!
Installation
PyPI

To install CryptIt, run this command in your terminal:
This is the preferred method to install CryptIt, as it will always install the most recent stable release.
Source files
In case you downloaded or cloned the source code from GitHub or your own fork, you can run the following to install cameo for development:

Note: Don’t forget about ‘sudo’!
Basic Usage
Available command list:
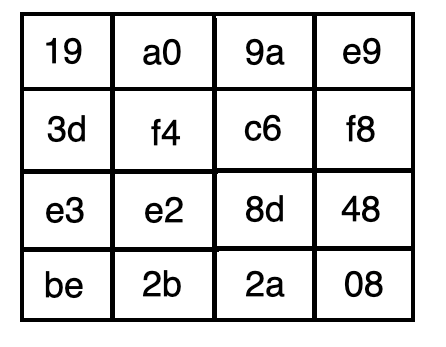
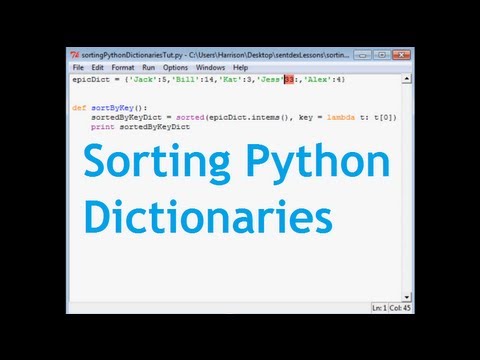
Encryption mode
To encrypt files on your PC open terminal and type following command, use -e option and put just path to target file or directory.
Bugs, issues and contributing
If you find bugs or have suggestions about improving the module, don’t hesitate to contact us.
License
This project is licensed under the MIT License - see the LICENSE file for details
Copyright (c) 2017 - Maxim Krivich, Ivan Kudryashov, Danil Naumenko
Release historyRelease notifications | RSS feed
0.1.6
0.1.5
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size cryptit-0.1.6-py2.py3-none-any.whl (11.7 kB) | File type Wheel | Python version py2.py3 | Upload date | Hashes |
Filename, size cryptit-0.1.6.tar.gz (8.7 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for cryptit-0.1.6-py2.py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | cf35393bae614fb615add47c859300f2a8dc8053448280c80278275a007096ff |
MD5 | 0364ff75034c093fe4f349f18fe0d1e9 |
BLAKE2-256 | 7d0cf7c53e03be8c8fc0eb9a826cd53f4c9dabfddd959525df771860c0db7eea |
Hashes for cryptit-0.1.6.tar.gz
Generate Aes 256 Key Python 3.7
Algorithm | Hash digest |
---|---|
SHA256 | 3b482d38f44ee15982d1fbabd55ff6107ee2a0ef4ad1163777cb5ec63dec19b0 |
MD5 | 5c8827889420e78fed416cb35124817d |
BLAKE2-256 | eadc7ff909fa17c168f5f322c4db239a997e9a34b86143d60f1fc552adee3f15 |
Generate Aes 256 Key Python 3.5
I'm trying to build two functions using PyCrypto that accept two parameters: the message and the key, and then encrypt/decrypt the message.
I found several links on the web to help me out, but each one of them has flaws:
This one at codekoala uses os.urandom, which is discouraged by PyCrypto.
Moreover, the key I give to the function is not guaranteed to have the exact length expected. What can I do to make that happen ?
Also, there are several modes, which one is recommended? I don't know what to use :/
Generate Aes 256 Key Python Download
Finally, what exactly is the IV? Can I provide a different IV for encrypting and decrypting, or will this return in a different result?
Generate 256 Bit Aes Key Python
Here's what I've done so far: